Hi! this is TDCIAN!
Let's find out about checking network connection status in SwiftUI!
Look at these codes!
(1) NetworkManager.swift
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import Foundation | |
import Network // Let's import Network | |
class NetworkManager: ObservableObject { | |
let monitor = NWPathMonitor() | |
let queue = DispatchQueue(label: "NetworkManager") | |
@Published var isConnected = true | |
// Image depends the network status. | |
var imageName: String { | |
return isConnected ? "wifi" : "wifi.slash" | |
} | |
// Text depends on the network status. | |
var connectionDescription: String { | |
if isConnected { | |
return "Internet connection looks good!" | |
} else { | |
return "It looks like you're not connected to the internet" | |
} | |
} | |
init() { | |
monitor.pathUpdateHandler = { path in | |
DispatchQueue.main.async { | |
self.isConnected = path.status == .satisfied | |
} | |
} | |
monitor.start(queue: queue) | |
} | |
// method working when you touch 'Check Status' button. | |
func startMonitoring() { | |
monitor.start(queue: queue) | |
monitor.pathUpdateHandler = { path in | |
self.isConnected = path.status == .satisfied | |
if path.usesInterfaceType(.wifi) { | |
print("Using wifi") | |
} else if path.usesInterfaceType(.cellular) { | |
print("Using cellular") | |
} | |
} | |
} | |
// method stop checking. | |
func stopMonitoring() { | |
monitor.cancel() | |
} | |
} |
(2) ContentView.swift
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import SwiftUI | |
struct ContentView: View { | |
@ObservedObject var networkManager = NetworkManager() | |
var body: some View { | |
Group { | |
if networkManager.isConnected { | |
// show main content | |
} else { | |
// show failed internet screen | |
} | |
} | |
ZStack { | |
Color(.systemBlue).ignoresSafeArea() | |
VStack { | |
Image(systemName: networkManager.imageName) | |
.resizable() | |
.scaledToFit() | |
.frame(width: 200, height: 200) | |
.foregroundColor(Color.white) | |
Text(networkManager.connectionDescription) | |
.font(.system(size: 18)) | |
.foregroundColor(.white) | |
.padding() | |
Button(action: { | |
networkManager.startMonitoring() | |
}, label: { | |
Text("Check Status") | |
.padding() | |
.font(.headline) | |
.foregroundColor(Color(.systemBlue)) | |
}) | |
.frame(width: 140) | |
.background(Color.white) | |
.clipShape(Capsule()) | |
.padding() | |
} | |
} | |
} | |
} |
(3) In simulator
When network is connected
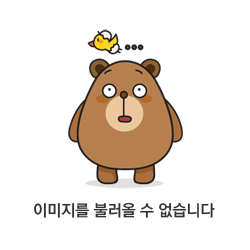
When network is not connected
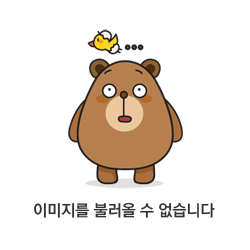
Full source code: https://github.com/TDCIAN/NetworkManagerSwiftUI
GitHub - TDCIAN/NetworkManagerSwiftUI: based on: https://www.youtube.com/watch?v=YIx3e0xWKtk
based on: https://www.youtube.com/watch?v=YIx3e0xWKtk - GitHub - TDCIAN/NetworkManagerSwiftUI: based on: https://www.youtube.com/watch?v=YIx3e0xWKtk
github.com
Reference
(1) https://www.youtube.com/watch?v=YIx3e0xWKtk
'iOS > SwiftUI' 카테고리의 다른 글
[iOS / SwiftUI] DownsizedImage (0) | 2025.03.03 |
---|---|
[iOS / SwiftUI] SwiftUI Notification Deep Linking (0) | 2025.02.16 |
[iOS / SwiftUI] SwiftUI Custom Alerts (0) | 2025.02.08 |
[iOS / SwiftUI] Use Deep Link in SwiftUI (0) | 2022.01.11 |
[iOS / SwiftUI] Bottom Sheet Drawer Use with Gesture (0) | 2022.01.07 |